Corso Algorithms and Data Structures in Python
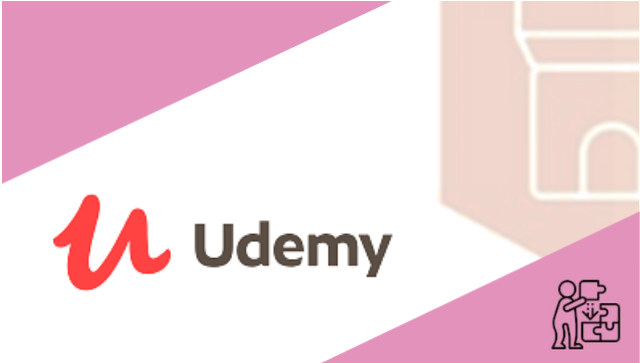
Course delivered in English
Lessons: 12
Level: Advanced
Access: Paid Online Course (price may vary)
A guide to implement data structures, graph algorithms and sorting algorithms from scratch with interview questions!
What you'll learn
- Understand arrays and linked lists
- Understand stacks and queues
- Understand tree like data structures (binary search trees)
- Understand balances trees (AVL trees and red-black trees)
- Understand heap data structures
- Understand hashing, hash tables and dictionaries
- Understand the differences between data structures and abstract data types
- Understand graph traversing (BFS and DFS)
- Understand shortest path algorithms such as Dijkstra's approach or Bellman-Ford method
- Understand minimum spanning trees (Prims's algorithm)
- Understand sorting algorithms
- Be able to develop your own algorithms
- Have a good grasp of algorithmic thinking
- Be able to detect and correct inefficient code snippets
This course includes:
- 18 hours on-demand video
- 33 articles
- 1 downloadable resource
- Full lifetime access
- Access on mobile and TV
- Certificate of completion
Requirements
- Python basics
- Some theoretical background ( big O notation )
Description
This course is about data structures, algorithms and graphs. We are going to implement the problems in Python programming language. I highly recommend typing out these data structures and algorithms several times on your own in order to get a good grasp of it.
So what are you going to learn in this course?
Section 1:
- setting up the environment
- differences between data structures and abstract data types
Section 2 - Arrays:
- what is an array data structure
- arrays related interview questions
Section 3 - Linked Lists:
- linked list data structure and its implementation
- doubly linked lists
- linked lists related interview questions
Section 4 - Stacks and Queues:
- stacks and queues
- stack memory and heap memory
- how the stack memory works exactly?
- stacks and queues related interview questions
Section 5 - Binary Search Trees:
- what are binary search trees
- practical applications of binary search trees
- problems with binary trees
Section 6 - Balanced Binary Trees (AVL Trees and Red-Black Trees):
- why to use balanced binary search trees
- AVL trees
- red-black trees
Section 7 - Priority Queues and Heaps:
- what are priority queues
- what are heaps
- heapsort algorithm overview
Section 8 - Hashing and Dictionaries:
- associative arrays and dictionaries
- how to achieve O(1) constant running time with hashing
Section 9 - Graph Traversal:
- basic graph algorithms
- breadth-first
- depth-first search
- stack memory visualization for DFS
Section 10 - Shortest Path problems (Dijkstra's and Bellman-Ford Algorithms):
- shortest path algorithms
- Dijkstra's algorithm
- Bellman-Ford algorithm
- how to detect arbitrage opportunities on the FOREX?
Section 11 - Spanning Trees (Kruskal's and Prim's Approaches):
- what are spanning trees
- what is the union-find data structure and how to use it
- Kruskal's algorithm theory and implementation as well
- Prim's algorithm
Section 12 - Sorting Algorithms
- sorting algorithms
- bubble sort, selection sort and insertion sort
- quicksort and merge sort
- non-comparison based sorting algorithms
- counting sort and radix sort
In the first part of the course we are going to learn about basic data structures such as linked lists, stacks, queues, binary search trees, heaps and some advanced ones such as AVL trees and red-black trees.. The second part will be about graph algorithms such as spanning trees, shortest path algorithms and graph traversing. We will try to optimize each data structure as much as possible.
In each chapter I am going to talk about the theoretical background of each algorithm or data structure, then we are going to write the code step by step in Python.
Most of the advanced algorithms relies heavily on these topics so it is definitely worth understanding the basics. These principles can be used in several fields: in investment banking, artificial intelligence or electronic trading algorithms on the stock market. Research institutes use Python as a programming language in the main: there are a lot of library available for the public from machine learning to complex networks.
Who this course is for:
- Beginner Python developers curious about graphs, algorithms and data structures
This course is added onto our catalogue to help you to understand the basics of Machine Learning, Data Science and Algorithms. Learning this topic opens up opportunities to be able to understand the role of a AI MAchine Learning and Big Data Specialist.
This course is managed by the external partner Udemy. In order to take this course you will need to register on their Academy platform where you can take advantage of this and many other courses on offer. This course however is not a free course but it is considered an excellent valuable asset to make you better yourself.
Once you have obtained your certification, save it on your device in Jpg or Pdf format and go back to the course page, in Guilds42, to upload it to the appropriate box and validate your skills.